DobotControl.py 예제 파일 소스코드 설명
□ Dobot, "Dobot Magician Demo Description", issue V1.1, 3/12/2019. 24-26 page DobotControl.py 프로그램 전문
import threading
import DobotDllType as dType
CON_STR = {
dType.DobotConnect.DobotConnect_NoError: "DobotConnect_NoError",
dType.DobotConnect.DobotConnect_NotFound: "DobotConnect_NotFound",
dType.DobotConnect.DobotConnect_Occupied: "DobotConnect_Occupied"}
# Load Dll and get the CDLL object
api = dType.load()
# Connect Dobot
state = dType.ConnectDobot(api, "", 115200)[0]
print("Connect status:",CON_STR[state])
if (state == dType.DobotConnect.DobotConnect_NoError):
# Clean Command Queued
dType.SetQueuedCmdClear(api)
# Async Motion Params Setting
dType.SetHOMEParams(api, 200, 200, 200, 200, isQueued = 1)
dType.SetPTPJointParams(api, 200, 200, 200, 200, 200, 200, 200, 200, isQueued = 1)
dType.SetPTPCommonParams(api, 100, 100, isQueued = 1)
# Async Home
dType.SetHOMECmd(api, temp = 0, isQueued = 1)
# Async PTP Motion
for i in range(0, 5):
if i % 2 == 0:
offset = 50
else:
offset = -50
lastIndex = dType.SetPTPCmd(api, dType.PTPMode.PTPMOVLXYZMode, 200 + offset, offset, offset, offset, isQueued = 1)[0]
# Start to Execute Command Queue
dType.SetQueuedCmdStartExec(api)
# Wait for Executing Last Command
while lastIndex > dType.GetQueuedCmdCurrentIndex(api)[0]:
dType.dSleep(100)
# Stop to Execute Command Queued
dType.SetQueuedCmdStopExec(api)
# Disconnect Dobot
dType.DisconnectDobot(api)
1.8 Python Demo
1.8.1 Project Description
There are two files in Python demo.
- DobotControl.py: Secondary encapsulation of Dobot API
- DobotDllType.py: Specific implementing file
Before running DobotControl.py, please add Dobot DLLs directory to the running directory of python, or add them to system environment variable.
1.8.2 Python API
DobotDllType.py encapsulates the C type interface of Dobot DLL, which is Python API of Dobot. The example for loading DLL is shown as follows.
[Program 1.73 Load DLL]
def load():
if platform.system() == "Windows":
print("your dll is 32 bit, in order to run the program successfully, please make sure that your python is 32
bit too")
print("python environment is:",platform.architecture())
return CDLL("./DobotDll.dll", RTLD_GLOBAL)
elif platform.system() == "Darwin":
return CDLL("./libDobotDll.dylib", RTLD_GLOBAL)
elif platform.system() == "Linux":
return cdll.loadLibrary("libDobotDll.so")
NOTICE
Please be sure to add Dobot DLLs directory to system environment variable, to ensure that DLLs are loaded correctly. For details, please see 1.1.2 Usage.
1.8.3 Code Description
When calling APIs related to motion (PTP, Jog, etc.), queue mode is used in this demo. (1) Load DLLs and obtain Store object (api). When Python API is called, this object will be used.
[Program 1.74 Load DLL]
api = dType.load()
(2) Connect to Dobot Magician and print the connecting information. After the connection is successful, the related codes will be handled.
[Program 1.75 Connect to Dobot]
state = dType.ConnectDobot(api, "", 115200)[0]
print("Connect status:",CON_STR[state])
if (state == dType.DobotConnect.DobotConnect_NoError):
#Dobot interactive codes
dType.DisconnectDobot(api)
(3) Control the queue:
- Clear the queue.
- Start the queue.
- Stop the queue.
[Program 1.76 Queue control]
dType.SetQueuedCmdClear(api)
dType.SetQueuedCmdStartExec(api)
dType.SetQueuedCmdStopExec(api)
(4) Set the motion parameters.
[Program 1.77 Set the motion parameters]
dType.SetHOMEParams(api, 200, 200, 200, 200, isQueued = 1)
dType.SetPTPJointParams(api, 200, 200, 200, 200, 200, 200, 200, 200, isQueued = 1)
dType.SetPTPCommonParams(api, 100, 100, isQueued = 1)
(5) Download the PTP commands to the queue and obtain the index of the last command.
[Program 1.78 PTP movement]
for i in range(0, 5):
if i % 2 == 0:
offset = 50
else:
offset = -50
lastIndex = dType.SetPTPCmd(api,
dType.PTPMode.PTPMOVLXYZMode,
200 + offset,
offset,
offset,
offset,
isQueued = 1)[0]
(6) Wait for the last motion command to be completed.
[Program 1.79 Wait for the last command]
while lastIndex > dType.GetQueuedCmdCurrentIndex(api)[0]:
dType.dSleep(100)
Dobot Python 모듈(API) 참고 문서
□ Dobot, "Dobot Magician API Description", Issue: V1.2.2, 11/6/2018.
Support > Download Center > Dobot Magician > Development Protocol
- 페이지에서 Dobot Magician API Description 문서 파일을 다운로드한다.
- 이 문서는 파이썬에서 사용한 API를 서술하고 있으며, 예로 "DobotControl.py" 에서 사용한 setPTPCmd()의 설명은 다음과 같다(36page).
Prototype
|
int SetPTPCmd(PTPCmd *ptpCmd, bool isQueued, uint64_t
*queuedCmdIndex)
|
Description
|
Execute a PTP command. Please call this API after setting the related
parameters in PTP mode to make the Dobot move to the target point
|
Parameter
|
PTPCmd:
typedef struct tagPTPCmd {
uint8_t ptpMode; //PTP mode (0-9)
float x; //Coordinate parameters in PTP mode. (x,y,z,r)
can be set to Cartesian coordinate, joints
angle, or increment of them
float y;
float z;
float r;
}PTPCmd;
Details for ptpMode:
enum {
JUMP_XYZ, //JUMP mode, (x,y,z,r) is the target point in
Cartesian coordinate system
MOVJ_XYZ, //MOVJ mode, (x,y,z,r) is the target point in
Cartesian coordinate system
MOVL_XYZ, //MOVL mode, (x,y,z,r) is the target point in
Cartesian coordinate system
JUMP_ANGLE, //JUMP mode, (x,y,z,r) is the target point in
Joint coordinate system
MOVJ_ANGLE, //MOVJ mode, (x,y,z,r) is the target point in
Joint coordinate system
MOVL_ANGLE, //MOVL mode, (x,y,z,r) is the target point in
Joint coordinate system
MOVJ_INC, //MOVJ mode, (x,y,z,r) is the angle increment
in Joint coordinate system
MOVL_INC, //MOVL mode, (x,y,z,r) is the Cartesian
coordinate increment in Joint coordinate
Executing a PTP Command with the I/O Control
Table 1.65 Execute a PTP command with the I/O control
system
MOVJ_XYZ_INC, //MOVJ mode, (x,y,z,r) is the Cartesian
coordinate increment in Cartesian
coordinate system
JUMP_MOVL_XYZ, //JUMP mode, (x,y,z,r) is the Cartesian
coordinate increment in Cartesian
coordinate system
};
ptpCmd: PTPCmd pointer
isQueued: Whether to add this command to the queue
queuedCmdIndex: If this command is added to the queue, queuedCmdIndex
indicates the index of this command in the queue. Otherwise, it is invalid
|
Return
|
DobotCommunicate_NoError: The command returns with no error
DobotCommunicate_BufferFull: The command queue is full
DobotCommunicate_Timeout: The command does not return, resulting in a
timeout
|
2023 EDBLab
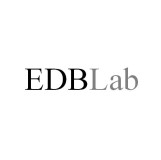
'로봇 프로그래밍' 카테고리의 다른 글
팔이동 - (4) Dobot Magician 파이썬 프로그래밍 (0) | 2023.03.30 |
---|---|
Dobot Magician 좌표 체계 및 동작 모드 (0) | 2023.03.29 |
모듈 파일 목록 - (3) Dobot Magician Python 프로그래밍 (0) | 2023.03.29 |
파이썬과 모듈 설치 - (1) Dobot Magician 파이썬 프로그래밍 (0) | 2023.03.27 |
Dobot Magician 에서 ERR_LIMIT_AXIS23_NEG 오류를 만났을 때 (0) | 2023.03.08 |